출처 : 컴공선배
HTTP 통신을 통해 networking을 하게 된다.
Rest API
GET 조회
POST 등록
PUT 업데이트
DELETE 삭제
URI : 자원까지 명시한 전체적인 주소
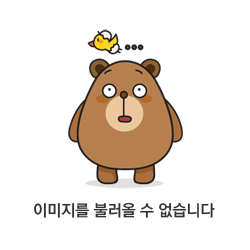
서버와의 연동은 비동기적인 작업이다
HttpURLConnection 을 편하게 하기 위해서 retrofit이 존재한다
Postman을 이용한 test
[실습]
build.gradle에 추가
//Retrofit
implementation "com.squareup.retrofit2:retrofit:2.9.0"
implementation "com.squareup.retrofit2:converter-gson:2.9.0"
implementation "com.squareup.retrofit2:adapter-rxjava2:2.9.0"
//okHttp
implementation "com.squareup.okhttp3:okhttp:4.9.0"
implementation "com.squareup.okhttp3:logging-interceptor:4.9.0"
//Glide
implementation 'com.github.bumptech.glide:glide:4.11.0'
annotationProcessor 'com.github.bumptech.glide:compiler:4.11.0'
manifest파일에 추가
<uses-permission android:name="android.permission.INTERNET"/>
User.kt data class에 @SerializedName(value="서버에서 설정한 키 값") 추가하기
@Entity(tableName = "UserTable")
data class User(
@SerializedName(value="email") var email : String,
@SerializedName(value="email") var password : String,
@SerializedName (value="email") var name: String
){
@PrimaryKey(autoGenerate = true) var id : Int = 0
}
AuthRetrofitInterface 설정
interface AuthRetrofitInterface {
@POST("/users")
fun signUp(@Body user: User): Call<AuthResponse>
}
AuthResponse 설정 : postman의 밑에 있는 부분 = 서버에서 응답하는 형태와 동일하게 data class를 추가
data class AuthResponse(val isSuccess:Boolean, val code:Int, val message: String)
NetworkModule 파일 생성 - retrofit 객체 생성
const val BASE_URL = "https://edu-api-test.softsquared.com" // 맨뒤에 /붙일거면 interface부분에서는 /빼주어야 함
//retrofit 객체 생성
fun getRetrofit(): Retrofit {
val retrofit = Retrofit.Builder().baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create()).build()
return retrofit
}
Activity에서의 사용
//retrofit 호출
val authService = getRetrofit().create(AuthRetrofitInterface::class.java)
//enqueue라는 메소드를 통해 응답 처리
authService.signUp(getUser()).enqueue(object : Callback<AuthResponse> {
//응답이 왔을 때 처리
override fun onResponse(call: Call<AuthResponse>, response: Response<AuthResponse>) {
Log.d("SIGNUP/SUCCESS",response.toString())
val resp: AuthResponse = response.body()!!
when (resp.code){
1000->finish()
2016, 2018 ->{
binding.signUpEmailErrorTv.visibility = View.VISIBLE
binding.signUpEmailErrorTv.text = resp.message
}
}
}
//연결 실패 시 실행
override fun onFailure(call: Call<AuthResponse>, t: Throwable) {
Log.d("SIGNUP/FAIL",t.message.toString())
}
})
실습 코드 모듈화
SignUpView라는 interface 생성 후 activity에서 상속 받기
AuthService라는 class 생성 후